Download demo
project - 36 Kb
The sample
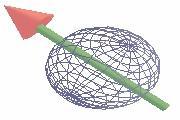
The sample
of the print preview
The article gives a class, CGLMemoryDC, which
can support OpenGL
printing under Win95/NT
CGLMemoryDC stores the DIB data for the
printer context device.
Collapse |
Copy
Code
private:
HBITMAP m_hBitmap; BITMAPINFO m_DIBInfo; BYTE* m_hImage; DWORD m_dwDataSize;
CGLMemoryDC also gives the functions to get
DIB data from selected context device or set DIB
data to target context device
Collapse |
Copy
Code
void CGLMemoryDC::CopyDataFromDC(CDC* pDC, CRect& rect)
{
CDC dcBuffer; CBitmap bmBitmap; CBitmap* pbmBitmapOld;
if(!m_hBitmap)
return;
dcBuffer.CreateCompatibleDC(pDC);
bmBitmap.CreateCompatibleBitmap(pDC,
m_DIBInfo.bmiHeader.biWidth,
m_DIBInfo.bmiHeader.biHeight);
pbmBitmapOld = dcBuffer.SelectObject(&bmBitmap);
dcBuffer.StretchBlt(0, 0,
m_DIBInfo.bmiHeader.biWidth,
m_DIBInfo.bmiHeader.biHeight,
pDC,
rect.left,
rect.top,
rect.Width(),
rect.Height(),
SRCCOPY);
dcBuffer.SelectObject(pbmBitmapOld);
GetDIBits(pDC->m_hDC,
HBITMAP)bmBitmap,
0,
m_DIBInfo.bmiHeader.biHeight,
m_hImage,
&m_DIBInfo,
DIB_RGB_COLORS);
}
void CGLMemoryDC::CopyDataToDC(CDC* pDC, CRect& rect)
{
::StretchDIBits(pDC->m_hDC,
rect.left,
rect.top,
rect.Width(),
rect.Height(),
0, 0,
m_DIBInfo.bmiHeader.biWidth,
m_DIBInfo.bmiHeader.biHeight,
m_hImage,
&m_DIBInfo,
DIB_RGB_COLORS,
SRCCOPY);
}
void CGLMemoryDC::WriteDataToDC(CDC* pDC, int startx, int starty)
{
::SetDIBitsToDevice(pDC->m_hDC,
startx,
starty,
m_DIBInfo.bmiHeader.biWidth,
m_DIBInfo.bmiHeader.biHeight,
0, 0, 0,
m_DIBInfo.bmiHeader.biHeight,
m_hImage,
&m_DIBInfo,
DIB_RGB_COLORS);
}
The function "WriteDataToDIBfile" in
CGLMemoryDC allow to store DIB data to disk file
The use of CGLMemoryDC in application program
to support printing is like
Collapse |
Copy
Code
void CGlprintView::OnDraw(CDC* pDC)
{
CGlprintDoc* pDoc = GetDocument();
ASSERT_VALID(pDoc);
if(pDC->IsPrinting())
{
CRect drawRect;
int cx, cy;
COLORREF clrOld = pDC->SetTextColor(RGB(250, 10, 10));
pDC->TextOut(450,10, "This is a demo of OpenGL print provided by Zhaohui Xing");
pDC->SetTextColor(RGB(128, 128, 255));
pDC->TextOut(40,80, "Large Size");
drawRect.SetRect(40, 160, 2440, 1960);
pDC->DPtoLP(&drawRect);
m_MemImageDC.CopyDataToDC(pDC, drawRect);
pDC->TextOut(40,1960, "Medium Size");
drawRect.SetRect(500, 2040, 2100, 3240);
pDC->DPtoLP(&drawRect);
m_MemImageDC.CopyDataToDC(pDC, drawRect);
pDC->TextOut(40,3260, "Orignal Size");
m_MemImageDC.GetMemorySize(&cx, &cy);
drawRect.SetRect(1000, 3400, 1000 + cx , 3400 + cy);
pDC->DPtoLP(&drawRect);
m_MemImageDC.CopyDataToDC(pDC, drawRect);
pDC->SetTextColor(clrOld);
}
else {
CPalette* oldPalette;
oldPalette = m_pDC->SelectPalette(&m_Palette, FALSE);
m_pDC->RealizePalette();
wglMakeCurrent(m_pDC->GetSafeHdc(), m_hRC);
DrawObject();
SwapBuffers(m_pDC->GetSafeHdc());
wglMakeCurrent(m_pDC->GetSafeHdc(), NULL);
CRect rect;
GetClientRect(&rect);
m_MemImageDC.SetMemorySize(rect.Width(), rect.Height());
m_MemImageDC.CopyDataFromDC(m_pDC, rect);
m_pDC->SelectPalette(oldPalette, FALSE);
}
}