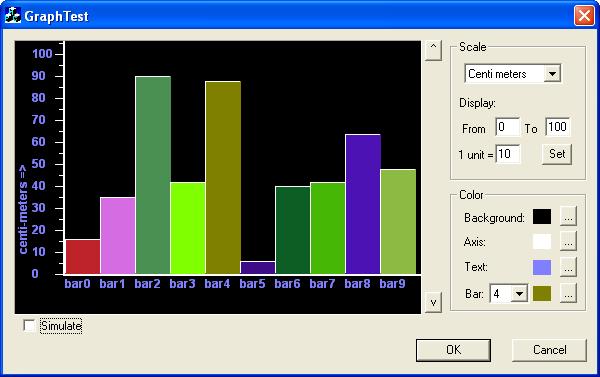
Introduction
Here is
a simple bar
graph control class derived
from
CStatic
.
It supports displaying
real-time data,
using any number of bars with different
colors, using a scale, both in
centimeter and inch, etc. The code uses
optimized plotting without flickering.
We can change the background color, the
text color, the
bar color etc., change the
scale, and change the display area.
Using
the code
-
Copy the files GraphCtrl.h
and GraphCtrl.cpp to your
project directory, and add to your
project.
-
Include GraphCtrl.h in
files where you need to use this
class.
-
Change
CStatic
definitions to
CGraphCtrl
.
- Use
the functions described below.
Methods
void
SetUnit(CString pUnit);
- Sets the unit type string to be
displayed near the Y axis.
void
SetScale(int);
- Sets the value of one unit in Y
axis.
int
GetScale();
- Returns the current scale value.
int
AddBar(int
iVal, COLORREF bClr,
const
char*
sLabel);
- Adds a new bar to the right end.
bClr
is the bar color and
sLabel
is the string to be displayed under
the bar.
int
InsertBar(int
index,int
iVal, COLORREF bClr,
const
char*
sLabel);
- Inserts a new bar at a position.
int
DeleteBar(int
index);
- Deletes a bar.
void
SetBarValue(int
index,
int iVal, BOOL bDraw=TRUE);
- Sets the value of a bar.
void
SetBarColor(int,
COLORREF, BOOL bDraw=TRUE);
- Sets the color of a bar.
int
GetBarValue(int
iIndex);
- Returns the value of the bar at a
position.
COLORREF GetBarColor(int
iIndex);
- Returns the color value of a bar
at a position.
void
SetBGColor(COLORREF clr);
- Sets the background color of the
graph.
COLORREF GetBGColor();
- Return the background color of the
graph.
void
SetAxisColor(COLORREF);
- Sets the color of the axis lines
in the graph.
COLORREF GetAxisColor();
- Returns the axis color.
void
SetTextColor(COLORREF);
- Sets the text color.
COLORREF GetTextColor();
- Returns the text color
int
GetNumberOfBars();
- Returns the number of bars.
int
DrawGraph(void);
- Draws
the graph.
int
SetDisplayRange(int
iRangeStart,
int
iRangeEnd);
- Sets the display range of the Y
axis.
void
GetDisplayRange(int& iRangeStart,
int& iRangeEnd);
- Gets the display range of the Y
axis.
Example
of use
Collapse
Copy Code
m_Graph.SetUnit("centi-meters");
m_Graph.SetScale(10);
m_Graph.GetDisplayRange(0, 100);
for(UINT i=0;i<10;i++)
{
char tmp[16];
sprintf(tmp, "bar%d", i);
m_Graph.AddBar(rand()%100, RGB(rand()%256, rand()%256, rand()%256), tmp);
sprintf(tmp, "%d", i);
m_BarCombo.AddString(tmp);
}
m_Graph.SetBGColor(RGB(0,0,0));
m_Graph.SetAxisColor(RGB(255,255,255));
m_Graph.SetTextColor(RGB(0,0,255));