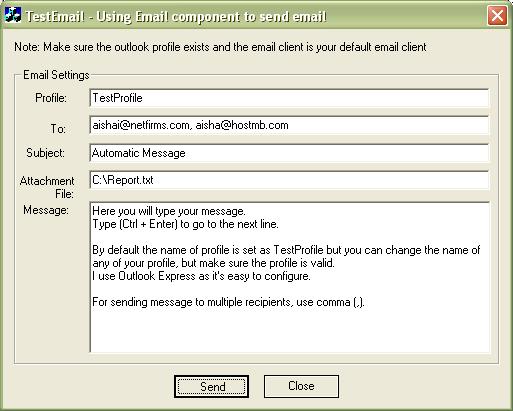
Introduction
During the software
development, I have seen many applications
that requires incorporating email
support. I remember, once I was to develop an
application, which sends emails
at the specific time to specific people with the
customized messages. For that I developed a COM service
that used MAPI. MAPI,
i.e. Messaging Application Programming
Interface, is the standard messaging architecture and a
complete set of functions and object-oriented
interfaces. Here I have an Email
component, a COM
DLL, which is a set of messaging functions that helps
you create messaging-enabled applications. This COM
component uses Simple MAPI
to achieve that.
Note: MAPI
is used by various industry-standard e-mail
clients, such as the Microsoft Exchange
client, all versions of Microsoft Outlook and Outlook
Express, including QUALCOMM Incorporated (Eudora) and
Netscape Communications Corporation. So you can use this
component with these client applications also.
Component
Design
The CLSID
of the Email component is CLSID_Mail
and it
has only one interface IMail
with the
interface ID IID_IMail
.
CMail
is
the implementation class and contains following data
members:
m_MAPILogon |
Function pointer
for MAPILogon |
m_MAPISendMail |
Function pointer
for MAPISendMail |
m_MAPISendDocuments |
Function pointer
for MAPISendDocuments |
m_MAPIFindNext |
Function pointer
for MAPIFindNext |
m_MAPIReadMail |
Function pointer
for MAPIReadMail |
m_MAPIResolveName |
Function pointer
for MAPIResolveName |
m_MAPIAddress |
Function pointer
for MAPIAddress |
m_MAPILogoff |
Function pointer
for MAPILogoff |
m_MAPIFreeBuffer |
Function pointer
for MAPIFreeBuffer |
m_MAPIDetails |
Function pointer
for MAPIDetails |
m_MAPISaveMail |
Function pointer
for MAPISaveMail |
IMail
have
the following functions:
IsMapiInstalled |
Checks if the MAPI
is installed on the system. It searches Win.INI
file for the MAPI
key. If found it returns S_OK. |
InitMapi |
After checking
the through IsMapiInstalled , it
initializes the MAPI
function pointers. Method should be called once
before using the component. |
put_strProfileName |
Takes the name
of the outlook profile name you are using to send
the email. |
put_strEmailAddress |
Sets the
recipient email address. You can specify more
than one comma (,) separated recipient
addresses. |
put_strRecipient |
Sets the name
you want to specify for the recipients, sets the
email names
of the sender – it may be different from your
specified profile email address. |
put_strSubject |
Sets the subject
of the email. |
get_strSubject |
Returns the email
subject. |
put_strMessage |
Sets email
message text. |
get_strMessage |
Returns message
text. |
put_strAttachmentFilePath |
Sets the email
attachment with the full path like “c:\abc.txt”.
|
get_strAttachmentFilePath |
Returns the path
of email attachment. |
put_strAttachmentFile |
The display name
of the attachment (Sample.txt), by
default, it’s the same as that specified in put_strAttachmentFilePath . |
get_strAttachmentFile |
Returns the
attachment file name. |
Logon |
Opens a new
logon session if not already opened, using
specified outlook profile, name and the profile
password, you must logon before sending the
email. I have set password to NULL
assuming that the profile you will specify have
NO password, but you can specify your own
password. Automatically called by Send
method. |
Logoff |
Logs off, and
closes the session. Automatically called by Send
method after sending
the email. |
Send |
Sends
the email,
requires valid outlook profile name, recipient
email address and a login session to send
email. |
Steps to execute the
demo project
The demo project
demonstrates the way you can use the component
to send an email.
In order to execute the demo project, the following
settings are required:
Step 1: You must have
some valid Output Express email
account or create one named TestProfile.
You can create an email
profile in Express from Tools>Accounts menu.
This will open Internet Accounts property sheet. On the
tab All, click the button Add and then Mail. A wizard
will let you create an email account, specify the valid
email address. For hotmail account, wizard automatically
sets the names of email servers.
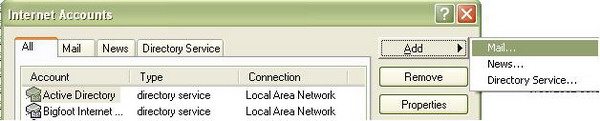
After successfully
creating the account, select the account name from the
list in All tab (for hotmail account, the default
account name is Hotmail). Open account properties by
pressing Properties button and change the account name
from default name (say Hotmail) to TestProfile.
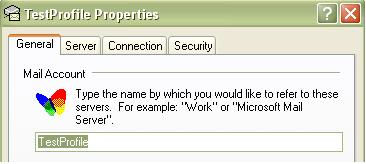
Step 2: Register the
DLL
All COM
DLLs required to be registered. After copying the DLL
source code, you can register the DLL by
right clicking the DLL and selecting Register DLL or Register
COM component option,
or simply by double clicking the DLL. Compiling the DLL
code in Visual Studio will automatically register the
DLL.
Step 3: Logged on to
the net
Make sure you are
logged on to the net. If not, outlook will fail to
deliver the email, however, you can still check the
composed email in
your outbox.
How to send
email?
After specifying the
information in the dialog box, click the Send button. A
warning message will be displayed, click Send button.
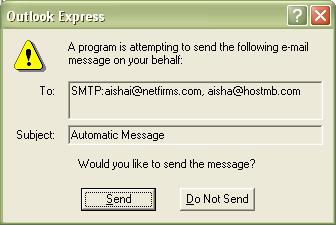
Note:The warning
dialog is displayed because of the security constraints.
If you want to get rid of this dialog box, then you will
have to use Extended MAPI
instead of simple MAPI.
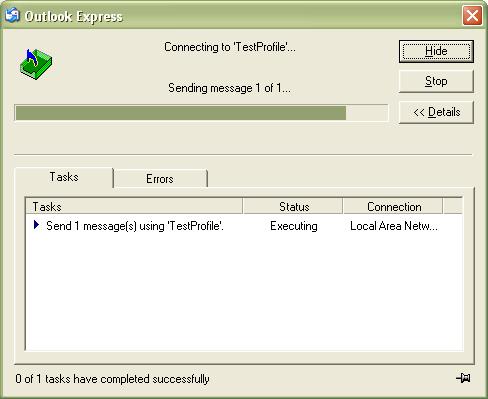
Here is the method CTestEmailDlg::OnSend
method, which is used to pass the user specified
information to the email object and call the IMail::Send
method after setting everything.
Collapse
//##//##//////////////////////////////////////////////////////////////
///////////////////////////////////////////////
//
// void CTestEmailDlg::OnSend()
//
// This module is called when send button is pressed and uses
//Email COM DLL to send
email - Aisha Ikram
// Note: dont forget to initialize COM library using CoInitialize(NULL);
//##//##////////////////////////////////////////////////////////////////////
///////////////////////////////////////////////
void CTestEmailDlg::OnSend()
{
UpdateData();
try{
CComPtr
objMail;
HRESULT hr;
// make sure the DLL is registered
hr = objMail.CoCreateInstance(CLSID_Mail);
if(SUCCEEDED(hr))
{
if(hr== S_OK)
{
// profile name is compulsory, this is the outlook profile,
// i used "outlook express" as configuring it is easier than
// "MS outlook" make sure to specify the correct sender's address
// for this profile and make sure that outlook express is
//the default email client.
if(m_strProfile.IsEmpty())
{
AfxMessageBox("Please specify email profile name ");
return;
}
if(m_strTo.IsEmpty())
{
AfxMessageBox("Please specify recipient's email address ");
return;
}
// by default, it's TestProfile, assumes that a profile with this
//name exists in outlook
hr= objMail->put_strProfileName((_bstr_t)m_strProfile);
hr = objMail->put_strSubject((_bstr_t)m_strSubject);
// this is the email or set of email addresses (separated by ,)
// which is actually used to send email
hr = objMail->put_strEmailAddress((_bstr_t)m_strTo);
// recipient is just to show the display name
hr = objMail->put_strRecipient((_bstr_t)m_strTo);
hr = objMail->put_strAttachmentFilePath((_bstr_t)m_strAttachment);
hr = objMail->put_strMessage((_bstr_t)m_strMessage);
hr= objMail->Send();
if(hr!=S_OK)
AfxMessageBox("Error, make sure the info is correct");
}//if
} //if
} // try
catch(...)
{
AfxMessageBox("Error, make sure specified info is correct");
}
}
Check the mail
in the recipient inbox.

Where to use
This component
can be extended to incorporate the functionalities like
opening an existing inbox to read emails
automatically from the inbox and composing new emails
etc. It can be used to send emails automatically with
customized user messages and attachments to specific
people at particular time, especially while using some
exe servers or NT services.
That's it. If there is
any suggestions or comments you are most welcome. Your
rating would help me evaluate the standard of my
article.
Looking for a Class
instead of a COM component?
I have also build a
class CSMAPI
, to use simple MAPI to send
email, read and find a message and more is to
be added. You can find this class, which you can use
anywhere (either in win32, or MFC or ATL) and the demo
project in MFC.