It is very easy (once
you see how it is done) to place
combo-boxes, edit
boxes, progress
controls, etc. into
toolbars. Below are two examples
of this, in the first a ComboBox
is placed on
a toolbar, and in
the second a cluster of checkboxes
is added. In both cases the technique is the same:


Step 1: Place
a button on
the toolbar in the
spot where you want the control(s) to eventually be. YOU
MUST place a seperator on either side of the button!.
Give the button an easily remembered resource name such
as IDP_PLACEHOLDER2 in the example
below.
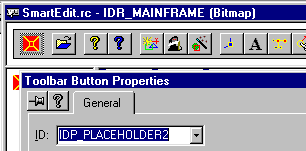
Step 2: Derive a
class from CToolBar
and give it a member variable for the control you will
be creating. For the ComboBox
example that class
looks like this. No extra methods are required, just a
place for the instance of the control to live.
class CMainToolBar : public CToolBar
{
public:
CComboBox m_wndSnap;
};
Step 3: In your
main frame's .h file replace the instance of the CToolBar
with you new class. Be sure to add an include statement
for the class definition created in step 1.
protected:
CStatusBar m_wndStatusBar;
CMainToolBar m_wndToolBar;
Step 4: At the
end of your main frame's OnCreate method you replace the
placeholder button with your control as follows:
Collapse
int SMCMainFrame::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
#define SNAP_WIDTH 80 //the width of the combo box
index = 0;
while (m_wndToolBar.GetItemID(index) != IDP_PLACEHOLDER2) index++;
m_wndToolBar.SetButtonInfo(index, IDP_PLACEHOLDER2, TBBS_SEPARATOR,
SNAP_WIDTH);
m_wndToolBar.GetItemRect(index, &rect);
rect.top+=2;
rect.bottom += 200;
if (!m_wndToolBar.m_wndSnap.Create(WS_CHILD|WS_VISIBLE|CBS_AUTOHSCROLL|
CBS_DROPDOWNLIST|CBS_HASSTRINGS,
rect, &m_wndToolBar, IDC_SNAP_COMBO))
{
TRACE0("Failed to create combo-box\n");
return FALSE;
}
m_wndToolBar.m_wndSnap.ShowWindow(SW_SHOW);
m_wndToolBar.m_wndSnap.AddString("SNAP OFF");
m_wndToolBar.m_wndSnap.AddString("SNAP GRID");
m_wndToolBar.m_wndSnap.AddString("SNAP RASTER");
m_wndToolBar.m_wndSnap.AddString("SNAP VERTEX");
m_wndToolBar.m_wndSnap.AddString("SNAP LINE");
m_wndToolBar.m_wndSnap.SetCurSel(0);
}
The result looks like
this:

Here is one that is a
little trickier:
Four check
boxes are placed in the toolbar.
In addition to adding multiple controls in place of a
single button this example
shows how to change the font of the checkboxes.

1. Derive the new
toolbar class and add it to the main frame. Also add a
CFont called gSmallFont to the Main Frame.
class CCoupleToolBar : public CToolBar
{
public:
CButton m_wndCenter;
CButton m_wndEdge;
CButton m_wndTrack;
CButton m_wndZoom;
};
2. Place
a placeholder button on
the toolbar resource
MAKING SURE to leave a space on either side. This is
done just as in the first example.
3. At the end of
OnCreate in the main frame, first set up the font we are
going to use, then replace the placeholder button with
the new controls.
Collapse
int CMainFrame::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
CClientDC DC(GetDesktopWindow());
long logical_pixels = DC.GetDeviceCaps(LOGPIXELSX);
if(logical_pixels <100)
{
gSmallFont.CreatePointFont(67, "DEFAULT", NULL);
}
else
{
gSmallFont.CreatePointFont(50, "DEFAULT", NULL);
}
#define CHECK_WIDTH 94
int index;
CRect rect;
CRect safe_rect;
index = 0;
while (m_wndViewBar.GetItemID(index) != IDP_PLACHOLDER) index++;
m_wndViewBar.SetButtonInfo(index, IDP_PLACHOLDER, TBBS_SEPARATOR,
CHECK_WIDTH);
m_wndViewBar.GetItemRect(index, &rect);
safe_rect=rect;
rect.left +=2;
rect.right=rect.left + ((CHECK_WIDTH / 2)-4);
rect.top=2;
rect.bottom=rect.top + 10;
if (!m_wndViewBar.m_wndCenter.Create("CNTR",
BS_CHECKBOX|WS_CHILD|WS_VISIBLE, rect, &m_wndViewBar,
IDM_COUPLE))
{
TRACE0("Failed to create CENTER check-box\n");
return FALSE;
}
m_wndViewBar.m_wndCenter.SendMessage(WM_SETFONT,
(WPARAM)HFONT(gSmallFont),TRUE);
rect.top = rect.bottom += 2;
rect.bottom = rect.top + 10;
if (!m_wndViewBar.m_wndEdge.Create("EDGE",
BS_CHECKBOX|WS_CHILD|WS_VISIBLE, rect, &m_wndViewBar,
IDM_COUPLE_EDGE))
{
TRACE0("Failed to create EDGE check-box\n");
return FALSE;
}
m_wndViewBar.m_wndEdge.SendMessage(WM_SETFONT,
(WPARAM)HFONT(gSmallFont), TRUE);
rect = safe_rect;
rect.left += ((CHECK_WIDTH / 2) + 4);
rect.top = 2;
rect.bottom = rect.top + 10;
if (!m_wndViewBar.m_wndZoom.Create("ZOOM",
BS_CHECKBOX|WS_CHILD|WS_VISIBLE, rect, &m_wndViewBar,
IDM_LOCK_ZOOMS))
{
TRACE0("Failed to create ZOOM check-box\n");
return FALSE;
}
m_wndViewBar.m_wndZoom.SendMessage(WM_SETFONT,
(WPARAM)HFONT(gSmallFont),TRUE);
rect.top = rect.bottom += 2;
rect.bottom = rect.top + 10;
if (!m_wndViewBar.m_wndTrack.Create("TRKR",
BS_CHECKBOX|WS_CHILD|WS_VISIBLE, rect, &m_wndViewBar,
IDM_SHOW_TRACKING))
{
TRACE0("Failed to create EDGE check-box\n");
return FALSE;
}
m_wndViewBar.m_wndTrack.SendMessage(WM_SETFONT,
(WPARAM)HFONT(gSmallFont), TRUE);
}