Multiple Monitor Support
Whilst working
on a screensaver for a client I came across
the problem of
multiple
monitors. No, not myself as I
am too poor to have two
monitors
on one system. However the client has a PC
set up grand enough to make Cape Canaveral
quake in its cowboy boots and run like a
buffalo.
For those of
you not aware of the problems of
multiple monitors
let me explain. Say we take Mr. Monitor
here. He uses points to provide a location
on his screen. Using an X and a Y
co-ordinate like ( 20, 100 ). These points
are taken relative to (0,0) which is the
very top-left hand corner of the screen.
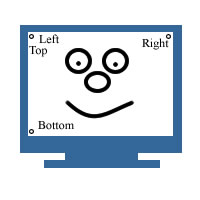
This is fine,
however when creating a screen saver you
need to know the boundaries that the screen
saver can go up to. There is no point in
making a screen saver that over-flows the
screen. So for getting the screen parameters
you may use this:
Collapse
Copy Code
CRect rect;
Rect.left = 0;
Rect.top = 0;
Rect.right = rect.left + ::GetSystemMetrics(SM_CYSCREEN);
Rect.bottom = rect.top + ::GetSystemMetrics(SM_CXSCREEN);
This code will
provide the bottom and right hand boundaries
as well as defining the top and left as
zero. From this you can create your window
size using this:
Collapse
Copy Code
const char* myWndClass = AfxRegisterWndClass(CS_HREDRAW|CS_VREDRAW|
CSSAVEBITS|CSDBLCLKS, NULL);
CWnd::CreateEx(WS_EX_TOPMOST, myWndClass, " ", WS_POPUP | WS_VISIBLE,
rect.left, rect.top, rectWidth(), rect.Height(), hwndParent, NULL );
If anyone using
a multiple monitor
system views the app with this
code they will only see the screensaver on
their primary monitor and will view the
desktop on the others. For this we need to
include a header file and change a little
bit of code.
The header file
is multimon.h and is available from
Microsoft. Or in the source zip on this
page. Add Multimon.h to your project
and add the line:
Collapse
Copy Code
#include "multimon.h"
to your Window
class source file.
Then we change
the code from:
Collapse
Copy Code
CRect rect;
Rect.left = 0;
Rect.top = 0;
Rect.right = rect.left + ::GetSystemMetrics(SM_CYSCREEN);
Rect.bottom = rect.top + ::GetSystemMetrics(SM_CXSCREEN);
To
Collapse
Copy Code
CRect rect;
Rect.left = ::GetSystemMetrics(SM_XVIRTUALSCREEN);
Rect.top = ::GetSystemMetrics(SM_YVIRTUALSCREEN);
Rect.right = rect.left + ::GetSystemMetrics(SM_CYVIRTUALSCREEN);
Rect.bottom = rect.top + ::GetSystemMetrics(SM_CXVIRTUALSCREEN);
That's it! The
screensaver will now run over the entire
virtual desktop.