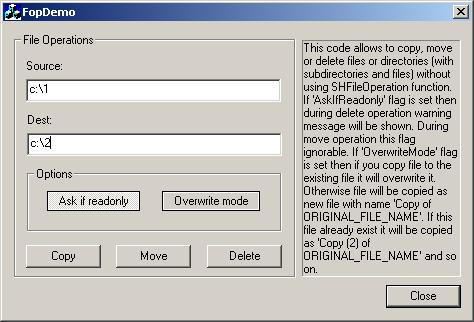
Introduction
There are no
methods in
VS for
copying
files
and
directories
(with
subdirectories
and
files)
based on API
functions.
It's
necessary to
use
SHFileOperation
function
from shell
to do it.
Sometimes
it's
inconvenient
and this
code is
designed to
fill this
space.
Background
This code is
a simple
wrapper over
API
functions
that allow
file
and dir
operations.
Recursion
methods are
the base of
this code.
MFC
was used for
simplification
of process
(only
CString
and
CFileFind
classes used
from). If
you don't
want to use
MFC
in your
project you
can change
MFC calls to
API calls
(use STL
string
and API
functions
FindFirstFile
and
FindNextFile
instead of
CFileFind
class).
Features
-
OverwriteMode
is set:
If you
copy
file
to the
existing
file
or to
the
folder
where
exist
file
with the
same
name, it
will
overwrite
it.
-
OverwriteMode
is not
set: If
you
copy
file
to the
existing
file
or to
the
folder
where
exist
file
with the
same
name, it
will
create
new file
with
name
'Copy of
ORIGINAL_FILE_NAME'.
If file
'Copy of
ORIGINAL_FILE_NAME'
already
exists
too, it
will
create
new file
with
name
'Copy
(2) of
ORIGINAL_FILE_NAME'
and so
on.
-
ucancode.netIfReadonly
is set:
If you
try to
delete
file
with
readonly
attribute
the
warning
message
will be
shown.
During
'replace'
operation
this
flag is
ignored.
-
ucancode.netIfReadonly
is not
set: If
you try
to
delete
file
with
readonly
attribute
it will
delete
without
any
question.
-
Path
presentation:
It is
unimportant
how you
represent
the path
with '\'
on end
or
without
it. For
example
you can
set
'c:\\1'
or
'c:\\1\\'
it's the
same.
You can
copy
file
to file,
file to
folder
or
folder
to
folder.
Just set
the
source
path and
destination
path.
Using the
code
-
Add
files
FileOperations.cpp
and
FileOpearations.h
to your
project.
-
In the
file
where
you want
to use
this
class
add
#include
"FileOpearations.h"
-
Create
CFileOperation
object
and use
it.
Sample code
Collapse
Copy
Code
#include "stdafx.h"
#include "FileOperations.h"
CFileOperation fo; fo.SetOverwriteMode(false); if (!fo.Copy("c:\\source", "c:\\dest")) {
fo.ShowError(); }
fo.Setucancode.netIfReadOnly(); if (!fo.Delete("c:\\source")) {
fo.ShowError(); }
If some
operation
failed you
can get
error code
or error
string or
show error
message (see
functions,
GetErrorCode()
,
GetErrorString()
and
ShowError()
accordingly).
For more
information
you can see
demo
project.
Available
methods
-
bool
Delete(CString
sPathName);
-
bool
Copy(CString
sSource,
CString
sDest);
-
bool
Replace(CString
sSource,
CString
sDest);
-
bool
Rename(CString
sSource,
CString
sDest);
-
CString
GetErrorString();
-
DWORD
GetErrorCode();
-
void
ShowError();
-
void
Setucancode.netIfReadOnly(bool
bucancode.net =
true);
-
bool
Isucancode.netIfReadOnly();
-
void
SetOverwriteMode(bool
bOverwrite
=
false);
-
bool
IsOverwriteMode();
-
bool
IsAborted();