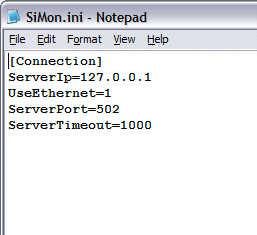
Introduction
I mostly use
INI files
to store
program
settings.
For this, I
use a
properties
class very
often.
Lately, I
optimized it
a little bit
and I
thought that
maybe
somebody
else could
also benefit
from this
class.
The
properties
class reads
the
INI file
when its
object is
created.
When no
INI file
exists, it
creates it
in the same
location as
where the
main
application
exe is
located. The
INI file
is named the
same as the
main exe but
with the
.ini
extension.
When the
object is
destroyed,
the
destructor
makes sure
that the
data is
stored in
the
INI file.
The reason I
use
INI files
more than
the registry
is because
when I have
to give
support, it
is easier
for the end
user to mail
me the
INI file
with all the
settings
than have
him look
into the
registry.
Sometimes
end users
are able to
make changes
to the
settings in
the
INI file
themselves.
Having users
editing the
registry is
more
dangerous
than editing
an
INI file
in my
opinion.
Using the
code
To use this
class, you
have to add
these lines
to the
stdafx.h:
Collapse
Copy
Code
#include "Properties.h"
extern CProperties prop;
Then add
this line to
the main
application
CPP file (myapp.cpp,
for example)
under the
line "CMyApp
theApp;
":
Collapse
Copy
Code
CProperties prop;
Adding data
to the
INI file
is easy.
Just add a
public
member of
type
CString
,
int
,
or
double
to the
header of
CProperties
(properties.h)
and then add
a line like
the
following in
the
constructor
of
CProperties
(properties.cpp):
Collapse
Copy
Code
class CProperties
{
public:
CString m_serverIp;
...
CProperties::CProperties()
{
m_proplist.push_back(
t_props("Connection", "ServerIp", "127.0.0.1", &m_serverIp));
^ ^ ^ ^
key name ___|value name __|default val __| data member __|
...
To compile
the
properties
class, add
the
properties.(cpp/h)
and
inifile.(cpp/h)
files to
your
project.
To access a
property
from your
source code,
you can type
something
like this.
Collapse
Copy
Code
void MyFunction()
{
CString server = prop.m_serverIp;
prop.m_serverIp = "10.0.0.1"; }