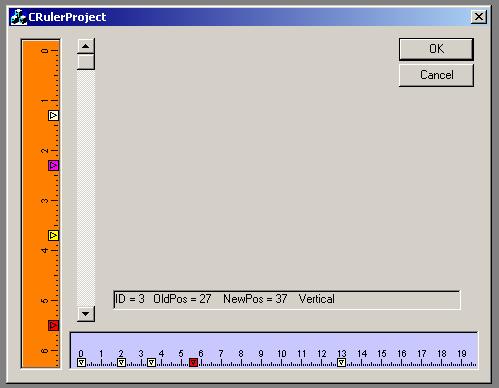
Introduction
This
control
is derived
from
MFC's
CWnd
class. While
you are
adding this
to your
dialogbox,
you should
select
"custom
control",
whose class
name should
be
CRulerWnd,
else, you
can't create
this
control.
This control
can be
located in
any
dialogbox or
View. The
RegisterWindowClass()
member
function
will
register
this window
class to
Windows.
After that,
you will not
have any
problem when
you create
it. The
class will
be
unregistered
in the
destructor
member
function.
Details
Collapse
Copy
Code
#define RULERWINDOW_CLASSNAME _T( "CRulerWnd" )
WNDCLASS wndcls;
HINSTANCE hInst = AfxGetResourceHandle();
if ( !( ::GetClassInfo( hInst, RULERWINDOW_CLASSNAME , &wndcls ) ) )
{
wndcls.style = CS_DBLCLKS | CS_HREDRAW | CS_VREDRAW;
wndcls.lpfnWndProc = ::DefWindowProc;
wndcls.cbClsExtra = wndcls.cbWndExtra = 0;
wndcls.hInstance = hInst;
wndcls.hIcon = NULL;
#ifndef _WIN32_WCE_NO_CURSOR
wndcls.hCursor = AfxGetApp()->LoadStandardCursor(IDC_ARROW);
#else
wndcls.hCursor = 0;
#endif
wndcls.hbrBackground = (HBRUSH)( COLOR_3DFACE + 1 );
wndcls.lpszMenuName = NULL;
wndcls.lpszClassName = RULERWINDOW_CLASSNAME;
if( !AfxRegisterClass( &wndcls ) )
{
AfxThrowResourceException();
return FALSE;
}
}
You can
select your
window
styles what
you want.
For
instance,
WS_EX_MODALFRAME
,
WS_EX_STATICEDGE
etc.
to create
for
miscellaneous
appearance.
This control
has a lot of
member
functions
for setting
it up. You
can use them
to change
colors and
other
things.
Collapse
Copy
Code
DWORD GetStyle() { return m_dwStyle; }
COLORREF GetBackGroundColor() { return m_clrBackGround; }
COLORREF GetMilimeterLineColor() { return m_clrMilimeterLineColor; }
COLORREF GetTextColor() { return m_clrTextColor; }
UINT GetStartSeperateSize() { return m_nSeperateSize; }
UINT GetMargin() { return m_nRulerMargin; }
UINT GetMilimeterPixel() { return m_nMilimeterPixel; }
UINT GetSeperatorSize() { return m_nSeperatorSize; }
long GetScrollPos() { return m_lScrolPos; }
CWnd* GetMessageTarget() { return m_pMessageTarget; }
BOOL SetStyle( DWORD dwStyle );
BOOL SetBackGroundColor( COLORREF clr );
BOOL SetMilimeterLineColor( COLORREF clr );
BOOL SetTextColor( COLORREF clr );
BOOL SetStartSeperateSize( UINT nSize );
BOOL SetMargin( UINT nMargin );
BOOL SetMilimeterPixel( UINT nPixel );
BOOL SetSeperatorSize( UINT nSize );
BOOL SetScrollPos( long lPos );
BOOL SetMessageTarget( CWnd *pTarget = NULL );
Additionaly,
you can add
a separator,
delete it,
change
position
etc.
Collapse
Copy
Code
SEPERATOR_TYPE* GetSeperator( int iID );
int DeleteAllSeperator();
int DeleteSeperator( int iID );
int AddSeperator( int iPos , int iID , int iType = 0 ,
LPARAM lParam = NULL ,
COLORREF clrLine = RGB( 0 , 0 , 0 ) ,
COLORREF clrFill = RGB( 255 ,255 , 220 ) ,
int iMinMargin = 0 , int iMaxMargin = 0xFFFFFFF );
For taking
separators,
use
CPtrArray
collection.
Collapse
Copy
Code
typedef struct _tagSEPERATOR_TYPE{
int iPos;
int iType;
int iID;
COLORREF clrLine;
COLORREF clrFill;
int iMinMargin;
int iMaxMargin;
LPARAM lParam;
}SEPERATOR_TYPE;
This
structure is
separate and
to the point
for each
separator
above.
Additionaly,
you can set
up each
separator
which can
have color,
position, id
number and
motion zone.
These are
shown as
below. All
notifications
can be sent
to whichever
window will
be the
target. This
notification
is as below:
Collapse
Copy
Code
#define NM_RULER_NOTIFICATIONMESSAGE 0x1112
#define NMSUB_RULER_SEPERATORCHANGE 0x0001
#define NMSUB_RULER_SEPERATORCHANGING 0x0002
typedef struct _tagRULERWNDNOTIFY_INFO{
NMHDR hdr;
UINT nSubMessage;
DWORD dwRulerStyle;
int iSepID;
int iNewPos;
int iOldPos;
int iParam1;
int iParam2;
}RULERWNDNOTIFY_INFO;