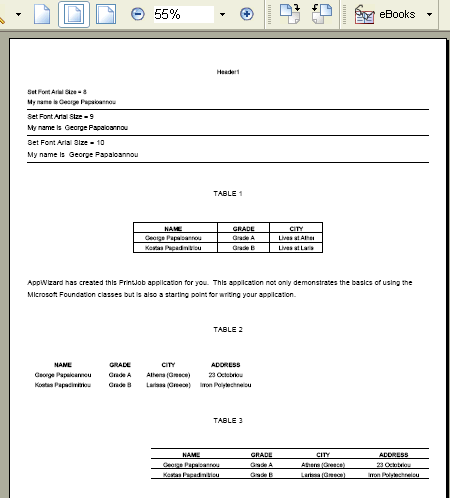
Introduction
This
printing
class
is based on
the
article
by Rob A.
Fraydl,
"Creating a
Printing
Class".
In fact,
it's just an
extension of
the original
class. What
exactly has
been
extended?
Well, first
of all, I
renamed the
class to
QPrint
(stands for
quick
print).
Big
achievement,
eh? OK, OK,
this feature
is not an
extension at
all, but
anyway it's
a start.
The second
extension
was to add
the ability
to manage
big strings
that extend
beyond the
right border
of the
physical
paper size.
Now, the big
strings are
not clipped
at the end
of the right
border, but
instead they
continue to
the next
line.
Last but not
the least,
the new
printing
class
supports
tables as
well as page
orientation.
Background
As already
said in the
beginning,
this piece
of code did
not start
from
scratch. You
can always
refer to the
original
printing
class named
CPrivatePrint
.
Please feel
free to use
and improve
this code.
Other than
the
additions I
have made, I
also
corrected
some bugs. I
am not sure
in some
points if
they were
bugs,
especially
the part
with the
logical
units, using
the macros
CALCF(x)
,
CALCY(y)
,
and
CALCX(x)
.
I deleted
CALCX(x)
completely,
and the
CALCY(x)
partially,
from the
source code.
So, if you
meet
"strange"
results,
please let
me know. The
others were
safe point
bugs that
got
corrected
successfully.
I tested
this class
using the
JawsPDF
Creator (to
save paper,
time,
patience,
and finally
money!) and
different
ink jet
printers. It
worked
flawlessly.
Using the
code
I chose this
printing
class
as a
base to
build
QuickPrint,
because it
was compact
and easy to
use. So, the
only thing
that you
have to do
is to
declare the
CQPrint prt
and start
using it.
The
following
example
shows the
basic steps
that you
have to do:
Collapse
Copy
Code
void TestDlg::OnPrintButton ()
{
CQPrint prt;
HPRIVATEFONT hFont;
if (prt.Dialog() == -1)
return;
prt.StartPrint();
hFont = prt.AddFontToEnvironment("Arial Greek",8,8);
prt.StartPage();
prt.Print(hFont,"Set Font Arial Size = 8",FORMAT_NORMAL);
prt.EndPage();
prt.EndPrint();
}
You can see
a live
example
(like the
picture in
this
tutorial) in
the
following
code:
Collapse
Copy
Code
CQPrint prt;
HPRIVATEFONT hFont,hFont1,hFont2;
CSize dim;
CRect margins;
if (prt.Dialog() == -1)
return;
prt.SetPageOrientation (DMORIENT_PORTRAIT);
prt.StartPrint();
hFont = prt.AddFontToEnvironment("Arial Greek",8,8);
hFont1 = prt.AddFontToEnvironment("Arial Greek",9,9);
hFont2 = prt.AddFontToEnvironment("Arial Greek",10,10);
prt.SetMargins(40,160,200,200);
prt.SetDistance(5);
prt.GetDrawDimension(dim);
prt.GetMargins(margins);
prt.SetHPos(dim.cy-margins.top);
prt.SetActiveFont (hFont);
prt.ActivateHF(HeaderFooter);
prt.StartPage();
prt.Print(hFont,"Set Font Arial Size = 8",FORMAT_NORMAL);
prt.Print(hFont,"My name is George Papaioannou",FORMAT_NORMAL);
prt.Line (PS_SOLID);
prt.Print(hFont1,"Set Font Arial Size = 9",FORMAT_NORMAL);
prt.Print(hFont1,"My name is George Papaioannou",FORMAT_NORMAL);
prt.Line (PS_SOLID);
prt.Print(hFont2,"Set Font Arial Size = 10",FORMAT_NORMAL);
prt.Print(hFont2,"My name is George Papaioannou",FORMAT_NORMAL);
prt.Line (PS_SOLID);
prt.lf(hFont2);
prt.lf(hFont2);
prt.Print(hFont2,"TABLE 1",FORMAT_CENTER);
prt.lf(hFont2);
prt.lf(hFont2);
int size[5];
size[0]=800;
size[1]=400;
size[2]=400;
size[3]=400;
prt.SetTableColumns (3);
prt.SetTableColumnsSize(size);
prt.SetTableHorLine (TRUE);
prt.SetTableVerLine (TRUE);
prt.SetTableBorderSize (200);
prt.SetTableJustify(FORMAT_CENTER);
prt.SetTableBorderLine(5);
prt.SetFace (hFont,FACE_NORMALBOLD);
prt.AddTableRecord (hFont,"NAME|GRADE|CITY",FORMAT_CENTER);
prt.SetFace (hFont,FACE_NORMAL);
prt.AddTableRecord (hFont,"George Papaioannou|Grade"
" A|Lives at Athens (Greece)",FORMAT_CENTER);
prt.AddTableRecord (hFont,"Kostas Papadimitriou|"
"Grade B|Lives at Larissa (Greece)",FORMAT_CENTER);
prt.lf(hFont2);
prt.lf(hFont2);
prt.Print(hFont2,"AppWizard has created this PrintJob"
" application for you. This application "
"not only demonstrates the basics"
" of using the Microsoft Foundation classes "
"but is also a starting point for"
" writing your application.",FORMAT_NORMAL);
prt.lf(hFont2);
prt.lf(hFont2);
prt.Print(hFont2,"TABLE 2",FORMAT_CENTER);
prt.lf(hFont2);
prt.lf(hFont2);
size[0]=800;
size[1]=400;
size[2]=600;
size[3]=600;
prt.SetTableReset();
prt.SetTableColumns (4);
prt.SetTableColumnsSize(size);
prt.SetFace (hFont,FACE_NORMALBOLD);
prt.AddTableRecord (hFont,"NAME|GRADE|CITY|ADDRESS",
FORMAT_CENTER);
prt.SetFace (hFont,FACE_NORMAL);
prt.AddTableRecord (hFont,"George Papaioannou|Grade A|"
"Athens (Greece)|23 Octobriou",FORMAT_CENTER);
prt.AddTableRecord (hFont,"Kostas Papadimitriou|Grade B|"
"Larissa (Greece)|Irron Polytechneiou",FORMAT_CENTER);
prt.lf(hFont2);
prt.lf(hFont2);
prt.Print(hFont2,"TABLE 3",FORMAT_CENTER);
prt.lf(hFont2);
prt.lf(hFont2);
size[0]=800;
size[1]=400;
size[2]=600;
size[3]=600;
prt.SetTableReset();
prt.SetTableColumns (4);
prt.SetTableColumnsSize(size);
prt.SetTableHorLine (TRUE);
prt.SetTableBorderSize (200);
prt.SetTableJustify(FORMAT_RIGHT);
prt.SetFace (hFont,FACE_NORMALBOLD);
prt.AddTableRecord (hFont,"NAME|GRADE|CITY|ADDRESS",FORMAT_CENTER);
prt.SetFace (hFont,FACE_NORMAL);
prt.AddTableRecord (hFont,"George Papaioannou|Grade A|"
"Athens (Greece)|23 Octobriou",FORMAT_CENTER);
prt.AddTableRecord (hFont,"Kostas Papadimitriou|Grade B|"
"Larissa (Greece)|Irron Polytechneiou",FORMAT_CENTER);
prt.EndPage();
prt.EndPrint();
You can call
the
following
table
methods:
CQPrint::SetTableColumns(int
Cols)
SetTableColumns
sets the
table
columns.
Collapse
Copy
Code
prt.SetTableColumns(3);
CQPrint::SetTableBorderSize(int
Border)
SetTableBorderSize
sets the
table
border
space.
Default is
50.
Collapse
Copy
Code
prt.SetTableBorderSize(50);
CQPrint::SetTableColumnsSize(int
Cols,int
size[])
SetTableColumnsSize
sets the
table column
size. The
maximum
number of
columns
permitted is
20.
Collapse
Copy
Code
int size[4];
size[0]=800;
size[1]=400;
size[2]=600;
size[3]=600;
prt.SetTableColumnsSize(4,size);
CQPrint::SetTableHorLine(BOOL
HLine)
SetTableHorLine
sets the
horizontal
lines.
Default is
OFF
(no lines).
Collapse
Copy
Code
prt.SetTableHorLine(TRUE);
CQPrint::SetTableVerLine(BOOL
HLine)
SetTableVerLine
sets the
vertical
lines.
Default is
OFF
(no lines).
Collapse
Copy
Code
prt.SetTableVerLine(TRUE);
CQPrint::SetTableBorderLine(int
Border)
SetTableBorderLine
sets the
width of the
border line.
Default is 0
(thin line).
Collapse
Copy
Code
prt.SetTableBorderLine(10);
CQPrint::SetTableJustify(int
Justification)
SetTableJustify
justifies
the table.
Default is
FORMAT_NORMAL
.
You can also
use
FORMAT_CENTER
and
FORMAT_RIGHT
.
Collapse
Copy
Code
prt.SetTableJustify(FORMAT_CENTER);
CQPrint::SetTableReset()
SetTableReset
resets all
the
table
parameters
to the
default
state. Use
this if you
don't want
to change
values when
using
consecutive
tables
with
different
visual
parameters.
Collapse
Copy
Code
prt.SetTableReset();
CQPrint::AddTableRecord(HPRIVATEFONT
font,
CString
strText,
UINT Format)
AddTableRecord
inserts
values to
the current
table. Use
the | symbol
to change
the column
in
strText
.
Collapse
Copy
Code
prt.AddTableRecord (hFont,"Column 1|Column 2|"
"Column 3|Column 4",FORMAT_CENTER);
If you want
to change
the page
orientation,
use
SetPageOrientation
before
StartPrint
.
Use the
values
DMORIENT_PORTRAIT
or
DMORIENT_LANDSCAPE
.
Collapse
Copy
Code
prt.SetPageOrientation (DMORIENT_PORTRAIT);
prt.StartPrint();
You can
always use
the
"original"
methods of
the class
like
SetMargins()
,
Line()
,
lf()
etc. More
details
about these
functions
can be found
in the demo.
You have to
experiment a
little
before you
use it.
Don't forget
that this
class also
supports
bitmap
printing
with the
InsertBitmap()
method. I
was
wondering if
there is
someone who
can extend
this class
to support
other image
formats
using GDI+.
Maybe the
next version
of
QPrint
will support
print
preview as
well as
image
printing (JPG,
GIF,
PCX
etc.), but
it's a
little bit
complicated,
since my
wife Natasa
is already
negative to
this
perspective.
So, don't
count on
this.
Don't forget
that you can
always use
the callback
function
ActivateHF()
that is used
to print
headers and
footers. I
have also
included
this feature
in my demo.
Anyway,
there are
more methods
in
QPrint
that you can
find in this
short
tutorial, so
start
playing with
the demo to
get an idea
of how this
stuff works,
and then try
to discover
the more
advanced
capabilities
that this
class
supports.
Points of
Interest
Please, if
you see
problems
with this
class,
especially
with the (LOGPIXELSY
,
LOGPIXELSX
)
width and
height of
the letters,
feel free to
contact with
me.