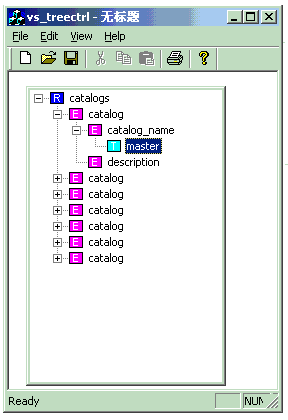
Introduction
When we are
typing in
Visual
Studio,
there is a
auto-completion
list for us
to complete
the
parameter
infomation
for the
function we
typed or
function
names for a
class. I
encountered
a
requirement
that the
user should
be able to
select some
words from a
tree
control
to complete
the current
place in the
an editor.
So I created
this
control.
How Does It
Work?
The control
is publicly
derived from
CTreeCtrl
.
You can
create it
using
CreateTree
with the
size, parent
window and
optional
bitmap
icons. When
you need to
display the
control,
simply use
the member
function
ShowMe
with default
parameters.
When you
double-click
on a tree
item, the
window of
the tree
control will
be closed
and it will
send a
message to
the parent
window with
selected
string.
Prototypes
Collapse
Copy
Code
class CXMLTreeCtrl : public CTreeCtrl
{
public:
BOOL CreateTree(CRect rect,CWnd *parent,UINT image=0);
void ShowMe(CPoint &pt,int show=SW_SHOW);
void SetImages(UINT resource);
BOOL loadXML(const CString &strPathName,
const BOOL bOptimizeMemory );
protected:
BOOL populateNode(MSXML::IXMLDOMElement* node, const HTREEITEM& hItem);
BOOL populateAttributes(MSXML::IXMLDOMElement *node, const HTREEITEM &hParent);
HTREEITEM insertItem(MSXML::IXMLDOMElement* node,
const CString &nodeName,
int nImage, int nSelectedImage,
HTREEITEM hParent = TVI_ROOT,
HTREEITEM hInsertAfter = TVI_LAST);
void deleteFirstChild(const HTREEITEM& hItem);
void deleteAllChildren(const HTREEITEM& hItem);
int getIconIndex(MSXML::IXMLDOMElement* node);
CImageList m_theImageList;
BOOL m_bOptimizeMemory;
protected:
afx_msg void OnItemexpanding(NMHDR* pNMHDR, LRESULT* pResult);
afx_msg void OnDblclk(NMHDR* pNMHDR, LRESULT* pResult);
afx_msg void OnLButtonUp(UINT nFlags, CPoint point);
How to use
it?
Using this
code
is very
simple.
First,
declare a
member
variable as
CXMLTreeCtrl
and
Create
it.
Collapse
Copy
Code
void CVs_treectrlView::OnInitialUpdate()
{
CView::OnInitialUpdate();
CRect rect(0,0,200,300);
m_Tree.CreateTree (rect,this,IDB_BITMAP_TREE_ICONS);
m_Tree.loadXML("catalog.xml",TRUE);
}
Secondly,
override the
WM_LBUTTONUP
or some
other
stimulus to
display the
tree
control
using
ShowMe
function.
Collapse
Copy
Code
void CVs_treectrlView::OnLButtonUp(UINT nFlags, CPoint point)
{
m_Tree.ShowMe (point);
CView::OnLButtonUp(nFlags, point);
}
Finally,
deal the
message
WM_FLOAT_CTRL
to do what
you have
selected
from tree
control.
Collapse
Copy
Code
LRESULT CVs_treectrlView::OnFloatCtrl(WPARAM wParam, LPARAM lParam)
{
CString str = (BSTR)wParam;
MessageBox(str);
m_Tree.ShowWindow (SW_HIDE);
return 0;
}
That's
all! Enjoy
it.