Video Capture with Visual C++ 2022
1
It is simple video capture application using
Windows form with Visual C++. This project
demonstrate the Visual C++ Configuration for
OpenCV as well as create windows form application.
OpenCV is the Open Computer Vision. It is open source library for image
processing. This article helps programmers to configure the visual studio for
visual c++ to run OpenCV application using
windows controls.
OpenCV library can be integrated into visual studio. It easy to configure.
Following six step shows the how to configure the visual studio 2010.
This article having the video capture project, which is made in Visual Studio
2010 version.
2
Prepare Visual Studio 2010
Step 1:
Go to Property Manager of project Select "Debug |
Win32" Right click on it. then Select
Properties then follow second step.
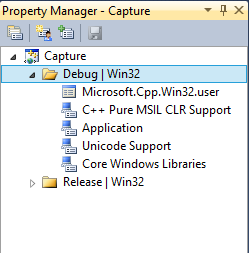
Step 2:
Select the General subcategory from C/C++ category. Select the Additional
Include Directories, add following path.
C:\opencv\build\include;
For 64bit :
C:\opencv\build\x86\vc10\bin ;
For
32bit :
C:\opencv\build\x64\vc10\bin ;
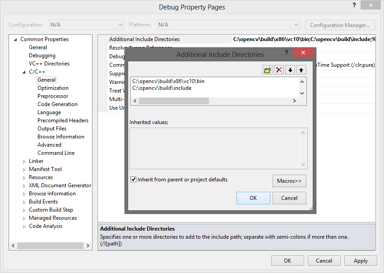
Step 3:
Set the library path to "C:\opencv\build\x86\vc10\lib" if the system is 64 bit,
else set library path to "C:\opencv\build\x64\vc10\lib". then click on Ok
button.
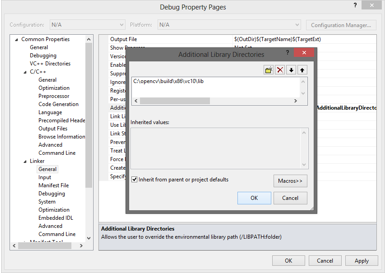
Step 4:
In this step, Need to add the OpenCV library. These are located at "C:\opencv\build\x86\vc10\lib"
path location.
For Debug
opencv_calib3d248d.lib |
opencv_contrib248d.lib |
opencv_core248d.lib |
opencv_features2d248d.lib |
opencv_flann248d.lib |
opencv_gpu248d.lib |
opencv_highgui248d.lib |
opencv_imgproc248d.lib |
opencv_legacy248d.lib |
opencv_ml248d.lib |
opencv_nonfree248d.lib |
opencv_objdetect248d.lib |
opencv_ocl248d.lib |
opencv_photo248d.lib |
opencv_stitching248d.lib |
opencv_superres248d.lib |
opencv_video248d.lib |
opencv_videostab248d.lib |
For
Release
Remove the last 'd' from file name. e.g. opencv_calib3d248d.lib
to opencv_calib3d248.lib
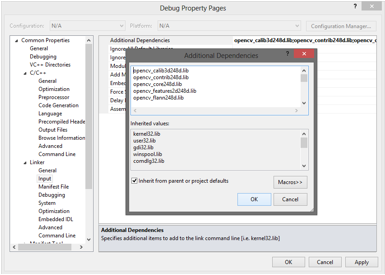
Step 5:
Change Common Language Runtime Support, Pure MSIL Common Language RunTime
Support (/clr:pure) to Common
Language RunTime Support (/clr). then click on "Ok" to finish configuration.
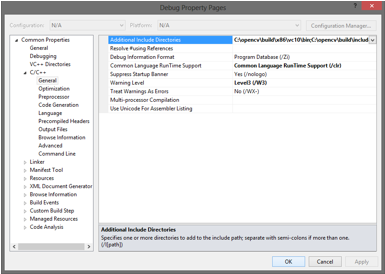
Step 6:
Add following Environmental Path.
For 64bit : C:\opencv\build\x86\vc10\bin ;
For 32bit : C:\opencv\build\x64\vc10\bin ;
3
Before going to write the code, need to include the header files as listed
follow. capture and frame variable declared
outside the namespace follow by the header files. These variable are the pointer
variables.
Collapse | Copy
Code
#pragma once
#include "opencv/cv.h"
#include "opencv/highgui.h"
#include "opencv2/opencv.hpp"
#include "opencv2/core/core.hpp"
CvCapture* capture;
IplImage* frame;
cvCaptureFromCAM function initialized the capture with first camera (index 0).
If index passed as 1 then, it initialized with second camera and so on.
Collapse | Copy
Code
capture = cvCaptureFromCAM(0);
Track bar initialized with total number of video frames. CV_CAP_PROP_FRAME_COUNT
is a constant which passed to cvGetCaptureProperty to get total number of frames
works with video file, not for cameras.
Collapse | Copy
Code
trackBar1->Maximum = (int)cvGetCaptureProperty(capture,CV_CAP_PROP_FRAME_COUNT);
Releases the capture from resources.
Collapse | Copy
Code
cvReleaseCapture(&capture);
Following function capture the
video from camera and also from
video file like *.avi, *.mp4 .
Collapse | Copy
Code
private: System::Void button2_Click(System::Object^ sender, System::EventArgs^ e)
{
if(comboBox1->Text == "")
{
MessageBox::Show(this,"Select Capture Method","Error!!!");
}
if(button2->Text == "Start")
{
if (comboBox1->Text == "Capture From Camera")
{
capture = cvCaptureFromCAM(0);
trackBar1->Minimum = 0;
trackBar1->Maximum = 0;
button2->Text = "Stop";
timer1->Start();
}
else if (comboBox1->Text == "Capture From File")
{
openFileDialog1->Filter = "AVI files (*.avi)|*.txt|All files (*.*)|*.*";
openFileDialog1->FilterIndex = 2;
openFileDialog1->RestoreDirectory = true;
openFileDialog1->FileName ="";
if ( openFileDialog1->ShowDialog() == System::Windows::Forms::DialogResult::OK )
{
char *fileName = (char*) Marshal::StringToHGlobalAnsi(openFileDialog1->FileName).ToPointer();
capture = cvCaptureFromFile(fileName);
trackBar1->Minimum = 0;
trackBar1->Maximum = (int)cvGetCaptureProperty(capture,CV_CAP_PROP_FRAME_COUNT);
button2->Text = "Stop";
timer1->Start();
}
}
}
else if(button2->Text == "Stop")
{
cvReleaseCapture(&capture);
button2->Text = "Start";
timer1->Stop();
}
}
cvQueryFrame function query the frame current video capture. and assigned to
frame variable.
Collapse | Copy
Code
frame = cvQueryFrame(capture);
Following statement is the replacement for the imshow( "windowname" , frame )
function of OpenCV which shows the image frame.
Collapse | Copy
Code
pictureBox1->Image = gcnew System::Drawing::Bitmap(frame->width,frame->height,frame->widthStep,System::Drawing::Imaging::PixelFormat::Format24bppRgb,(System::IntPtr) frame->imageData);
pictureBox1->Refresh();
Timer initialized with 30 intervals. It executes the function after 30 interval
of time. Following function Query the frame , and extract the video properties.
Following constants are used to extract video properties
CV_CAP_PROP_POS_FRAMES : Get current position of video
frame.
CV_CAP_PROP_FOURCC : Get video codec
information.
CV_CAP_PROP_POS_MSEC : Get Time information.
CV_CAP_PROP_FRAME_HEIGHT : Get video frame
height.
CV_CAP_PROP_FRAME_WIDTH : Get video frame
width.
CV_CAP_PROP_FPS : Get video frame rate (Fame
Per Seconds) .
Collapse | Copy
Code
private: System::Void timer1_Tick(System::Object^ sender, System::EventArgs^ e)
{
try
{
frame = cvQueryFrame(capture);
if(frame != NULL)
{
pictureBox1->Image = gcnew System::Drawing::Bitmap(frame->width,frame->height,frame->widthStep,System::Drawing::Imaging::PixelFormat::Format24bppRgb,(System::IntPtr) frame->imageData);
pictureBox1->Refresh();
trackBar1->Value = (int)cvGetCaptureProperty(capture,CV_CAP_PROP_POS_FRAMES);
double codec_double = cvGetCaptureProperty(capture,CV_CAP_PROP_FOURCC);
label6->Text = "Codec: " + System::Text::Encoding::UTF8->GetString(BitConverter::GetBytes((int)codec_double));
label7->Text = "Time: " + (TimeSpan::FromMilliseconds( cvGetCaptureProperty(capture,CV_CAP_PROP_POS_MSEC) ).ToString())->Substring(0, 8);
label8->Text = "Frame No.: " + (int)cvGetCaptureProperty(capture,CV_CAP_PROP_POS_FRAMES);
label9->Text = "Video Resolution: " + (int)cvGetCaptureProperty(capture,CV_CAP_PROP_FRAME_HEIGHT) + " X " + (int)cvGetCaptureProperty(capture,CV_CAP_PROP_FRAME_WIDTH);
label11->Text = "Video Frame Rate: " + (int)cvGetCaptureProperty(capture,CV_CAP_PROP_FPS);
}
}catch(...){}
}
Track Bar initialized with position of current video frame.
CV_CAP_PROP_POS_FRAMES is a constant which is used for getting the current
position of video frame.
Collapse | Copy
Code
private: System::Void trackBar1_Scroll(System::Object^ sender, System::EventArgs^ e)
{
cvSetCaptureProperty(capture,CV_CAP_PROP_POS_FRAMES, trackBar1->Value);
}